Skip to content
Java & Related Technologies
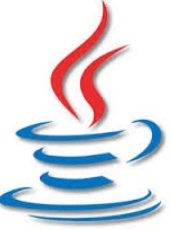
Java
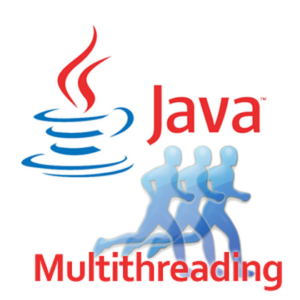
MultiThreading
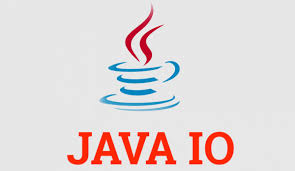
IO
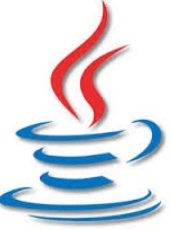
Collections
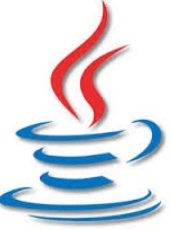
Date API
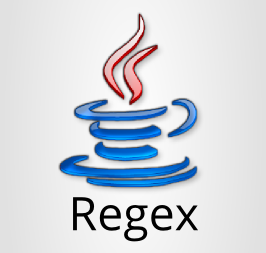
Regex
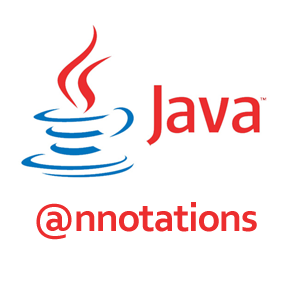
Annotations
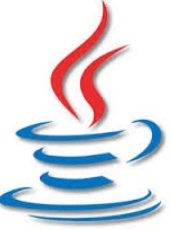
JavaMail
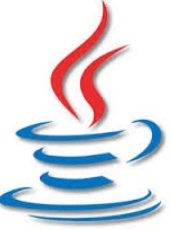
Design Principles
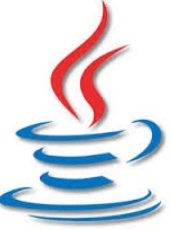
Design Patterns
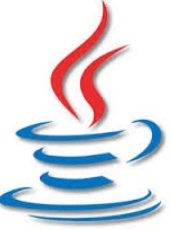
Java 7
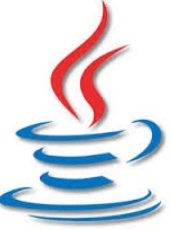
Java 8
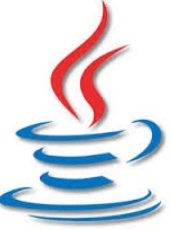
Servlet
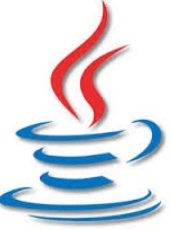
JSP
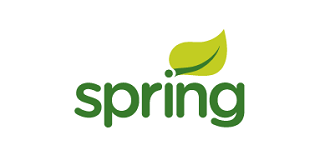
Spring Core
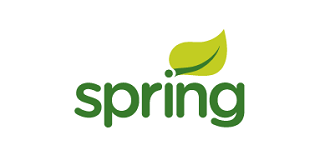
Spring AOP
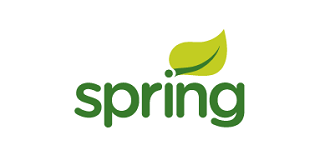
Spring DI
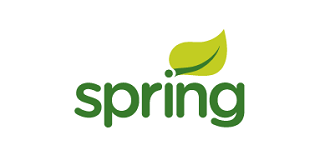
Spring MVC
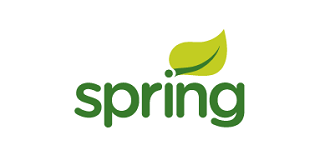
Spring SPEL
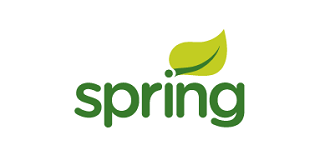
Spring Boot
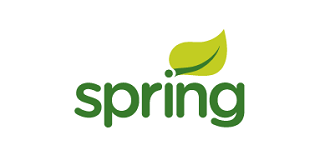
Spring Security

Hibernate
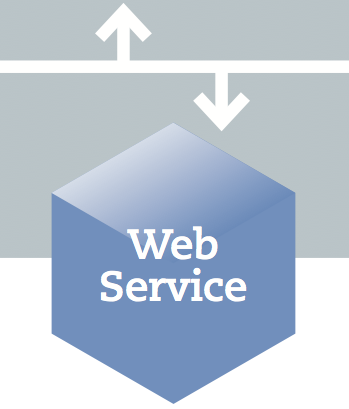
WebServices
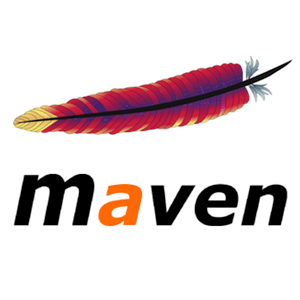
Maven
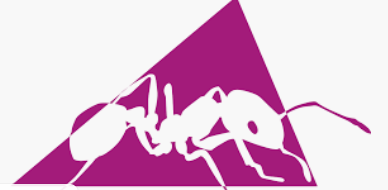
Ant
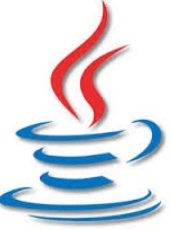
JAR
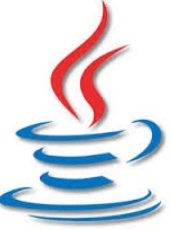
WAR
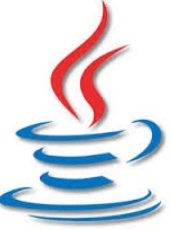
JDBC
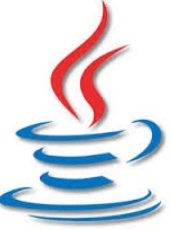
JPA
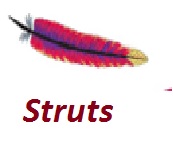
Struts2
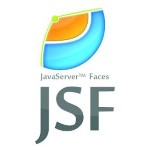
JSF
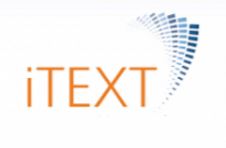
iText
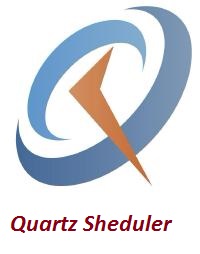
Quartz
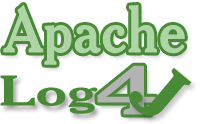
Log4j
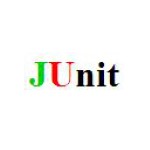
JUnit
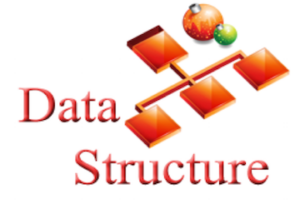
Java DS
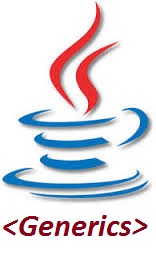
Generics
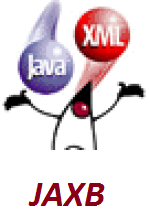
JAXB
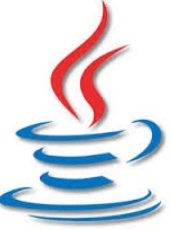
JSoup
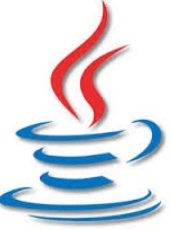
JSON
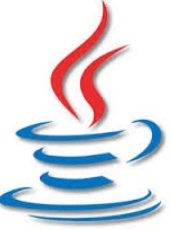
XML Parser
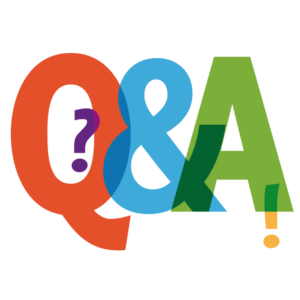
Q&A
Web Technologies
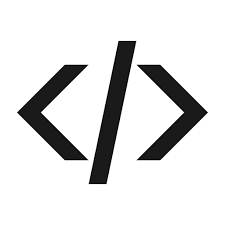
HTML
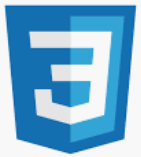
CSS
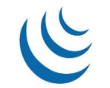
JQuery
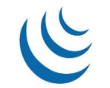
JQuery UI
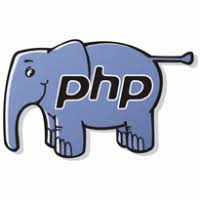
PHP
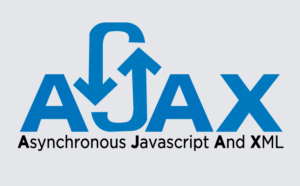
Ajax
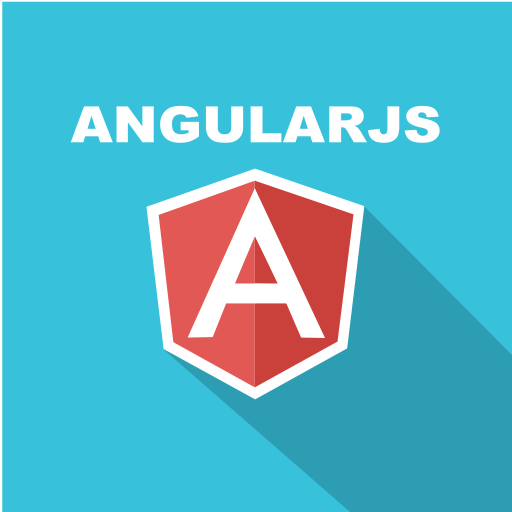
AngularJS
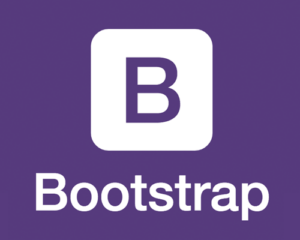
BootStrap
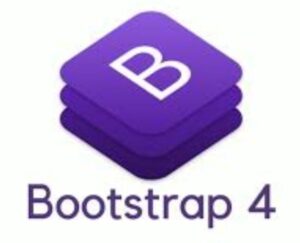
BootStrap 4
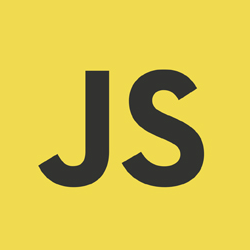
JavaScript
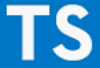
TypeScript
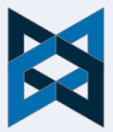
BackboneJS
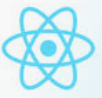
ReactJS
DataBase
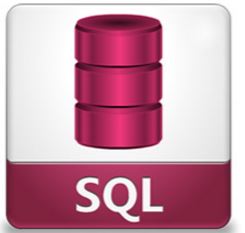
SQL
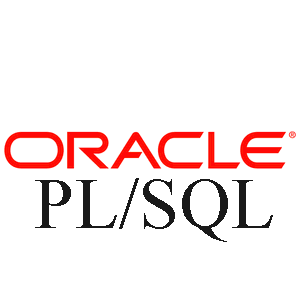
PLSQL
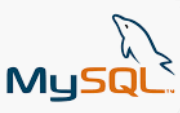
MySQL
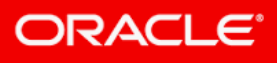
Oracle
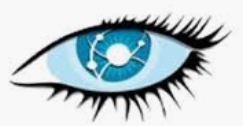
Cassandra
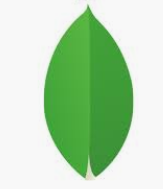
MongoDB
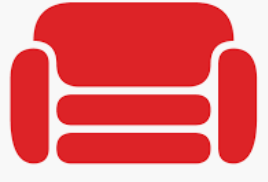
CouchDB
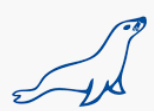
MariaDB
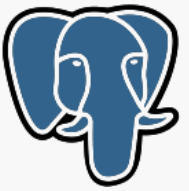
PostgreSQL
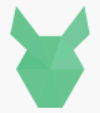
PouchDB
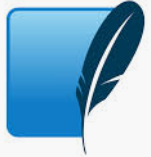
SQLite
Miscellaneous
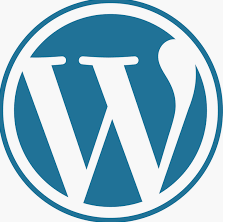
WordPress
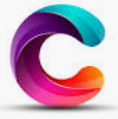
C
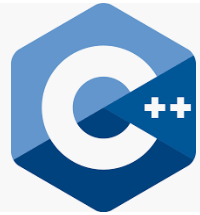
C++
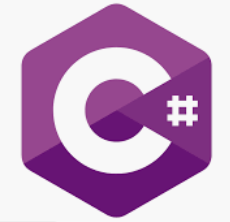
C#
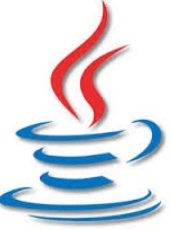
Python
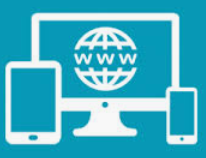
Computer Fundamentals
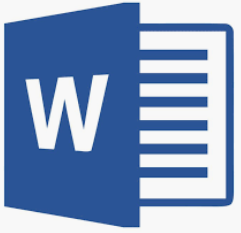
MS Word
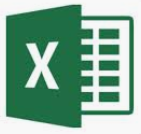
MS Excel
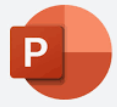
PowerPoint

Human Resource
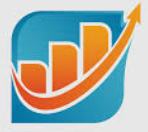
Marketing
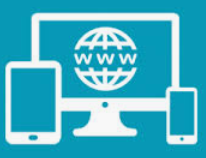
Stories
Academics
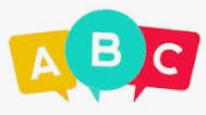
English
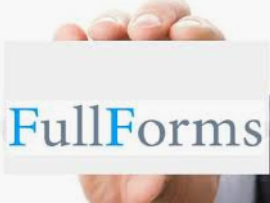
Full Form
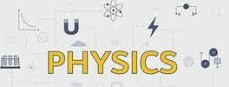
Physics
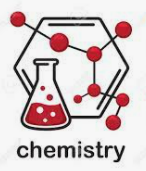
Chemistry
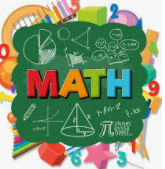
Math
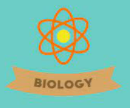
Biology
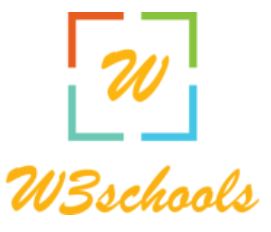
Stories